Hello Learners…
Welcome to the blog…
Topic: Top Python Interview Questions for Fresher Machine Learning Engineers
Table Of Contents
- Introduction
- Top Python Interview Questions for Fresher Machine Learning Engineers
- Summary
- References
Introduction
In this post, we discuss Top Python Interview Questions for Fresher Machine Learning Engineers.
Python is a popular programming language for machine learning engineers. It is easy to learn, has a large library of machine learning libraries, and is well-suited for data analysis.
Top Python Interview Questions for Fresher Machine Learning Engineers
Here are some Python-related questions that are commonly asked during interviews for fresher machine learning engineer positions,
- What is the difference between a list and a tuple in Python?
- How do you handle missing or null values in a Pandas DataFrame?
- What is the purpose of NumPy in Python? Give an example of how you would use it.
- Explain the concept of object-oriented programming (OOP) and provide an example of implementing a class in Python.
- How does a Python dictionary differ from a list or a tuple? Provide an example of when you would use a dictionary.
- What is the difference between shallow copy and deep copy in Python? How would you perform each type of copy?
- Explain the usage of the
lambda
function in Python. Provide an example of when you would use it. - How do you handle overfitting in machine learning models? Name some techniques used to prevent overfitting.
- What is the purpose of the
__init__
method in Python classes? How is it different from other methods? - Explain the concept of gradient descent in machine learning and its significance.
- What are functions and modules?
- How do you write loops and conditional statements?
- What are some of the most popular machine-learning libraries in Python?
- How do you load and save data in Python?
- How do you perform data visualization in Python?
- How do you debug Python code?
1. What is the difference between a list and a tuple in Python?
- A list is a mutable data structure, meaning its elements can be modified after creation.
- A tuple is an immutable data structure, and its elements cannot be changed once defined.
- Examples,
List:
# Example of a list (mutable)
fruits_list = ['apple', 'banana', 'orange']
fruits_list.append('grape')
fruits_list[1] = 'kiwi'
print(fruits_list) # Output: ['apple', 'kiwi', 'orange', 'grape']
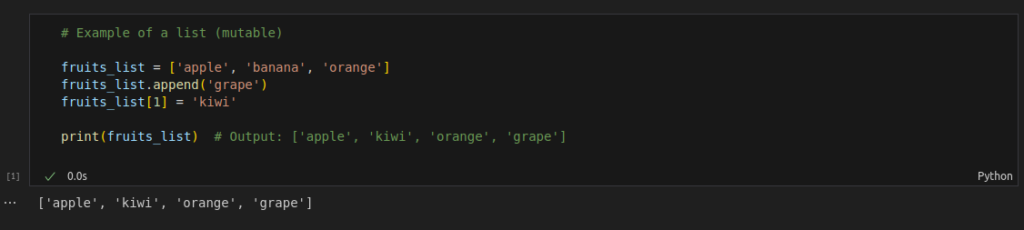
Tuple:
# Example of a tuple (immutable)
fruits_tuple = ('apple', 'banana', 'orange')
# fruits_tuple.append('grape') # Error: Tuples are immutable, so appending is not allowed
# fruits_tuple[1] = 'kiwi' # Error: Tuples are immutable, so element assignment is not allowed
print(fruits_tuple) # Output: ('apple', 'banana', 'orange')
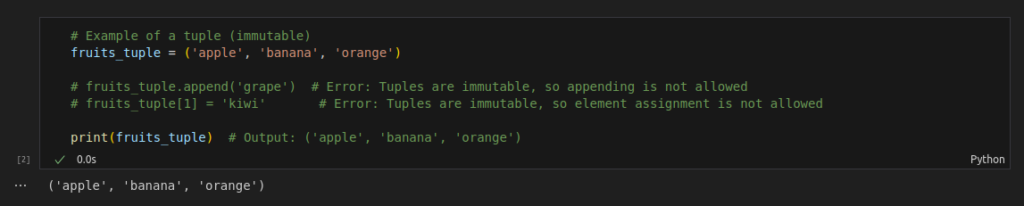
2. How do we handle missing or null values in a Pandas DataFrame?
There are many techniques using which we can handle missing or null values in Pandas DataFrame.
- Identifying missing values:
- The
isnull()
function returns a DataFrame with the same shape as the original, where each element is a boolean value indicating whether it’s a missing value or not. - The
notnull()
the function is the opposite ofisnull()
and returnsTrue
for non-null values.
- The
- Dropping missing values:
- The
dropna()
function allows you to remove rows or columns with missing values from the DataFrame. - Specify the
axis
parameter as 0 to drop rows with missing values, or as 1 to drop columns with missing values. - Additional parameters like
subset
andthresh
provide flexibility in defining conditions for dropping.
- The
- Filling missing values:
- The
fillna()
the function helps in replacing missing values with specified values. - You can pass a single value, such as
fillna(0)
, to replace all missing values with that value.
- The
Let’s see some examples,
import pandas as pd
import numpy as np
# Creating a sample DataFrame with missing values
data = {'Name': ['Ram', 'Ramesh', np.nan, 'Suresh'],
'Age': [25, 30, np.nan, 35],
'Salary': [50000, np.nan, 70000, np.nan]}
df = pd.DataFrame(data)
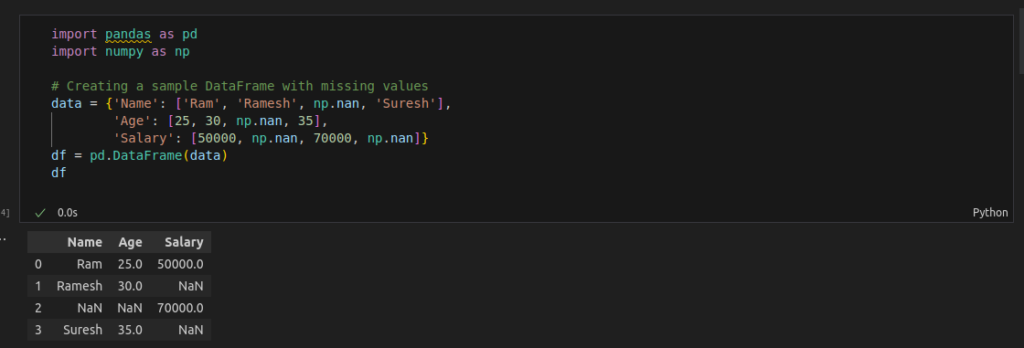
# Identifying missing values
print(df.isnull())
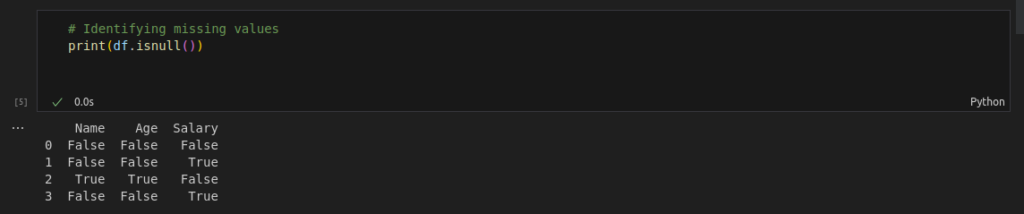
# Dropping missing values
df_dropped = df.dropna() # Drops rows with any missing values
print(df_dropped)

# Filling missing values
df_filled = df.fillna(0) # Replaces missing values with 0
print(df_filled)
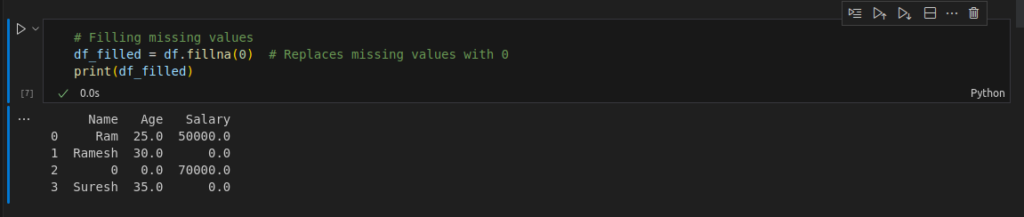
3. What is the purpose of NumPy in Python? Give an example of how we can use it.
NumPy, short for Numerical Python, is a powerful library in Python designed to facilitate numerical and scientific computations. It serves as a fundamental tool for handling large, multi-dimensional arrays and matrices, and provides a wide range of mathematical functions to efficiently operate on these arrays.
The primary purpose of NumPy is to enhance numerical computing capabilities in Python
import numpy as np
# Grades of students in a class
grades = [78, 85, 90, 92, 88, 79]
# Convert the grades to a NumPy array
grades_array = np.array(grades)
# Calculate the average grade using NumPy
average_grade = np.mean(grades_array)
# Print the average grade
print("The average grade is:", average_grade)
In this example, we import the NumPy library as np
and create a list of grades representing student performance. We convert the list into a NumPy array using np.array(), which allows us to apply mathematical operations easily. We then calculate the average grade using the np.mean()
function.
4. Explain the concept of object-oriented programming (OOP) and provide an example of implementing a class in Python.
object-oriented programming (OOP) is a programming paradigm that emphasizes the organization of code around objects, which are instances of classes
object-oriented programming (OOP) provides a structured and modular approach to designing and developing software by encapsulating data and the operations that manipulate that data within objects.
Let’s take an example of a class to illustrate the concept of OOP in Python:
class Car:
def __init__(self, brand, color):
self.brand = brand
self.color = color
def accelerate(self):
print(f"The {self.color} {self.brand} is accelerating.")
def brake(self):
print(f"The {self.color} {self.brand} is braking.")
# Creating objects (instances) of the Car class
car1 = Car("Toyota", "Red")
car2 = Car("Honda", "Blue")
# Accessing attributes and invoking methods
print(car1.brand) # Output: Toyota
print(car2.color) # Output: Blue
car1.accelerate() # Output: The Red Toyota is accelerating.
car2.brake() # Output: The Blue Honda is braking.
In this example, we define a Car
class that has attributes like brand
and color
. The __init__()
method serves as a constructor and is invoked when an object is created. It initializes the attributes of the object using the provided values.
The class also has methods like accelerate()
and brake()
that represent behaviors associated with a car. These methods can access the object’s attributes using the self
parameter, allowing manipulation of the object’s state or performing specific actions.
We then create two instances of the Car
class (car1
and car2
) with different brand and color values. We can access the attributes of these objects using dot notation (object.attribute
) and invoke their methods.
5. How does a Python dictionary differ from a list or a tuple? Provide an example of when we would use a dictionary.
Python dictionary is a data structure that stores key-value pairs, allowing efficient retrieval of values based on their associated keys. Unlike lists or tuples, which store elements sequentially, dictionaries provide a way to organize and access data using unique keys as identifiers.
Here’s an example to illustrate the difference between a dictionary, a list, and a tuple:
# Example of a dictionary
student = {
"name": "Ram",
"age": 20,
"major": "Computer Science"
}
# Example of a list
grades = [85, 90, 92, 88]
# Example of a tuple
coordinates = (10, 20)
In this example, we have a dictionary named student
that represents information about a student. The keys (“name”, “age”, “major”) serve as labels, and the corresponding values represent the actual data. Dictionaries provide a way to access values quickly by specifying the associated key, such as student["name"]
to retrieve the student’s name.
On the other hand, the grades
variable is a list that stores a sequence of numerical values representing the student’s grades. Lists are ordered and indexed, so we can access individual elements using their position, such as grades[0]
to retrieve the first grade.
The coordinates
variable is a tuple that stores an ordered pair of values. Tuples, like lists, are ordered, but unlike lists, tuples are immutable, meaning their elements cannot be modified after creation.
Dictionaries provide efficient lookup and retrieval of data, making them suitable for scenarios where quick access to values based on keys is required.
6. What is the difference between shallow copy and deep copy in Python? How would you perform each type of copy?
In Python, when we want to create a copy of an object, we have two options: shallow copy and deep copy.
Shallow Copy:
- A shallow copy creates a new object, but the content of the new object still references the same memory locations as the original object. In other words, the copy is a new container, but it still shares the underlying data with the original object. If changes are made to the original object’s data, those changes will be reflected in the copied object as well.
- To perform a shallow copy in Python, we can use the
copy()
method or the slice operator[:]
on the original object.
Here’s an example:
import copy
original_list = [1, 2, [3, 4]]
shallow_copy_list = copy.copy(original_list)
original_list[0] = 5
original_list[2][0] = 6
print(shallow_copy_list) # Output: [1, 2, [6, 4]]
In this example, we create a list original_list
with nested elements. We then perform a shallow copy using copy.copy()
and modifying the values of the original list. As a result, the modified values are also reflected in the shallow copy.
Deep Copy:
- A deep copy, on the other hand, creates a completely independent copy of the original object, including all of its nested objects. It means that any changes made to the original object or its nested objects will not affect the copied object, and vice versa.
To perform a deep copy in Python, we can use the deepcopy()
method from the copy
module. Here’s an example:
import copy
original_list = [1, 2, [3, 4]]
deep_copy_list = copy.deepcopy(original_list)
original_list[0] = 5
original_list[2][0] = 6
print(deep_copy_list) # Output: [1, 2, [3, 4]]
In this example, we perform a deep copy using copy.deepcopy()
and modify the values of the original list. However, the deep copy remains unchanged, demonstrating that it is a separate and independent copy.
7. Explain the usage of the lambda
function in Python. Provide an example of when you would use it.
lambda function in Python is a concise way to create anonymous functions. Unlike regular functions defined using the def
keyword, lambda functions are defined using the lambda
keyword and do not require a separate function name.
The lambda function syntax follows this format: lambda arguments: expression
. It can take any number of arguments, separated by commas, and consists of a single expression that is evaluated and returned as the result of the function.
Here’s an example to illustrate the usage of a lambda function:
# Example 1: Doubling a number using a lambda function
double = lambda x: x * 2
result = double(5)
print(result) # Output: 10
In this example, we define a lambda function called double
that takes a single argument x
and returns its doubled value. We then call the lambda function with the argument 5
, and the returned result is printed.
Lambda functions are particularly useful when we need to define small, one-time-use functions without the need for a formal function definition. They are commonly used in scenarios that require passing a function as an argument to another function or when working with higher-order functions like map()
, filter()
, or reduce()
.
Here’s an example demonstrating the usage of a lambda function with map()
to convert a list of integers to their squares:
# Example 2: Squaring a list of numbers using a lambda function with map()
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x ** 2, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
In this example, we use the map()
function along with a lambda function to apply the squaring operation to each element of the numbers
list. The lambda function takes an argument x
and returns the square of x
. The resulting squared numbers are then converted back into a list using list()
.
8. How do you handle overfitting in machine learning models? Name some techniques used to prevent overfitting.
overfitting is a common challenge in machine learning models where a model performs extremely well on the training data but fails to generalize well to unseen data. It occurs when a model learns the noise or random fluctuations in the training data, rather than capturing the underlying patterns or relationships.
To handle overfitting, various techniques can be employed. Let’s discuss a few commonly used techniques:
Cross-Validation:
- Cross-validation is a technique that helps assess a model’s performance on unseen data. It involves splitting the available data into multiple subsets, using one subset for validation while training the model on the rest. This allows for a more robust evaluation of the model’s generalization capabilities.
Regularization:
- Regularization is a technique that adds a penalty term to the loss function during training to discourage the model from overemphasizing complex patterns in the data. Common regularization methods include L1 regularization (Lasso), L2 regularization (Ridge), and Elastic Net regularization. These techniques introduce a trade-off between model complexity and the magnitude of the coefficients, promoting simpler models that generalize better.
Feature Selection:
- Overfitting can occur when the model is trained on irrelevant or noisy features. Feature selection methods help identify the most informative and relevant features for the model, discarding the ones that contribute less to the predictive performance. This reduces the complexity of the model and helps prevent overfitting.
Early Stopping:
- Training a model for too long can lead to overfitting. Early stopping is a technique where the training process is stopped before convergence based on a validation metric. By monitoring the validation performance, training is halted when further iterations no longer improve the model’s generalization ability, preventing overfitting.
Data Augmentation:
- Data augmentation involves artificially expanding the training dataset by applying various transformations or modifications to the existing data. This helps increase the diversity of the training samples, providing the model with a broader range of examples to learn from and reducing overfitting.
Ensemble Methods:
- Ensemble methods combine multiple models to make predictions. Techniques such as bagging (e.g., Random Forest) and boosting (e.g., Gradient Boosting) can be effective in reducing overfitting. By aggregating the predictions of multiple models, ensemble methods can compensate for the biases and errors of individual models, leading to improved generalization performance.
9. What is the purpose of the __init__
method in Python classes? How is it different from other methods?
the __init__
method in Python classes is a special method that is automatically called when an object is created from a class. It serves as a constructor or initializer for the object, allowing you to define the initial state and behavior of the object.
The __init__
method is different from other methods in a class in the following ways:
Object Initialization:
- The
__init__
method is responsible for initializing the object’s attributes and setting its initial state. It is called only once during object creation, right after the object is instantiated. Other methods in the class can be called multiple times throughout the object’s lifecycle.
Automatic Invocation:
- Unlike other methods that need to be explicitly called, the
__init__
method is automatically invoked when an object is created from the class. When you create an instance of a class using the class name followed by parentheses, likemy_object = MyClass()
, the__init__
method is executed, allowing you to perform any necessary setup or initialization.
Self-Reference:
- The
__init__
method always takes the first parameter namedself
. This parameter represents the instance of the class being created and is used to access and modify the object’s attributes and behavior. It allows you to store values specific to each instance and customize the object’s behavior based on its initial state.
Here’s an example to illustrate the usage of the __init__
method:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
# Create an instance of the Person class
person1 = Person("Ram", 25)
# Call the greet method of the person1 object
person1.greet() # Output: Hello, my name is Alice and I am 25 years old.
In this example, the __init__
method is defined in the Person
class. It takes two parameters, name
and age
, which are used to initialize the name
and age
attributes of the object. The greet
method is another method in the class that can be called on the object to display a personalized greeting.
10. Explain the concept of gradient descent in machine learning and its significance.
Gradient descent is an iterative optimization algorithm used to minimize the loss function or error of a machine learning model. It is commonly applied in various optimization problems, including training neural networks and fitting regression models.
The main idea behind gradient descent is to find the optimal values for the model’s parameters by iteratively updating them in the direction of the steepest descent of the loss function. It operates based on the gradient, which represents the rate of change of the loss function with respect to the model parameters.
Here’s a step-by-step explanation of the gradient descent process:
1. Initialization:
- The algorithm starts by initializing the model’s parameters with arbitrary values.
2. Forward Propagation:
- The input data is passed through the model to obtain predictions. These predictions are compared with the actual values to calculate the loss.
3. Backward Propagation:
- The gradients of the loss function with respect to the model parameters are computed using a technique called backpropagation. This involves calculating the partial derivatives of the loss function with respect to each parameter and tracing the impact of each parameter on the loss.
4. Parameter Update:
- The model parameters are updated by subtracting a fraction of the gradients from their current values. The fraction is determined by the learning rate, which controls the step size in each iteration.
5. Repeat:
- Steps 2 to 4 are repeated iteratively until a stopping criterion is met, such as reaching a maximum number of iterations or achieving a desired level of convergence.
The significance of gradient descent lies in its ability to optimize complex models with large numbers of parameters. It allows models to automatically adjust their parameters based on the training data, gradually reducing the loss and improving predictive performance. By iteratively updating the parameters in the direction of the steepest descent, gradient descent helps to find the global or local minimum of the loss function.
Some More Interview Questions for Fresher Machine Learning Engineers
11. What are functions and modules?
functions and modules are essential concepts in Python programming that help organize and reuse code effectively.
Functions:
- Functions in Python are named blocks of code that perform a specific task. They allow you to break down complex problems into smaller, more manageable tasks, making the code modular and easier to understand. Functions are defined using the
def
keyword, followed by the function name, parentheses, and a colon. They can take input parameters, perform operations, and optionally return a value.
Modules:
- In Python, a module is a file containing Python definitions, functions, and statements. It serves as a container to organize related code into a single file, making it easier to manage and reuse. Modules allow you to logically group related functionalities together, providing a structured way to organize and share code across different projects.
12. How do you write loops and conditional statements?
In Python, loops and conditional statements are used in Python to control the flow of execution and make decisions based on specific conditions.
Loops:
Loops allow us to repeatedly execute a block of code until a certain condition is met. In Python, there are two types of loops: for
loop and while
loop.
For Loop:
The for
loop is used when we know the number of iterations in advance. It iterates over a sequence (such as a list, tuple, or string) or any iterable object. The general syntax of a for
loop in Python is:
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print(fruit)
While Loop:
The while
loop is used when we don’t know the number of iterations beforehand, and the loop continues until a certain condition becomes False
. The general syntax of a while
loop in Python is:
count = 0
while count < 5:
print(count)
count += 1
13. What are some of the most popular machine-learning libraries in Python?
Python has a rich ecosystem of machine-learning libraries that provide powerful tools and algorithms to facilitate the development and implementation of machine-learning models. Here are some of the most popular machine-learning libraries in Python:
Scikit-learn:
- Scikit-learn is a widely used machine learning library that provides a comprehensive set of tools for various tasks, including classification, regression, clustering, dimensionality reduction, and model evaluation. It offers a user-friendly interface and supports a wide range of machine-learning algorithms.
TensorFlow:
- TensorFlow is an open-source library primarily focused on deep learning. It provides a flexible framework for building and training neural networks across different platforms and devices. TensorFlow offers a high-level API (Keras) as well as a lower-level API for more advanced customization.
PyTorch:
- PyTorch is another popular deep-learning library known for its dynamic computation graph and ease of use. It provides a flexible platform for building and training neural networks and has gained popularity in both academia and industry. PyTorch supports automatic differentiation and offers a Pythonic interface.
NumPy:
- Although not solely a machine learning library, NumPy is a fundamental package for scientific computing in Python. It provides support for efficient numerical operations and multi-dimensional array manipulations. Many other machine learning libraries, including TensorFlow and PyTorch, rely on NumPy for their underlying computations.
Pandas:
- Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames, which allow for efficient handling and processing of structured data. Pandas are often used for data preprocessing, exploration, and data wrangling tasks in machine learning pipelines.
SciPy:
- SciPy is a library that builds upon NumPy and provides a wide range of scientific computing and optimization tools. It includes modules for numerical integration, optimization, linear algebra, signal processing, and more. SciPy complements other machine learning libraries by offering additional functionality.
14. How do you load and save data in Python?
In Python, loading and saving data is a crucial aspect of working with Python for data analysis and machine learning. Python provides various libraries and methods to handle different types of data and file formats.
Here are some commonly used approaches for loading and saving data in Python:
CSV Files:
- CSV (Comma-Separated Values) files are a popular format for tabular data. To load data from a CSV file, we can use the
csv
module in Python’s standard library or libraries like Pandas.
Here’s an example using Pandas:
import pandas as pd
data = pd.read_csv('data.csv')
To save data to a CSV file using Pandas:
data.to_csv('new_data.csv', index=False)
JSON Files:
- JSON (JavaScript Object Notation) files are commonly used for storing and exchanging structured data. Python’s built-in
json
module makes it easy to load and save data in JSON format.
Here’s an example:
import json
# Loading data from a JSON file
with open('data.json') as file:
data = json.load(file)
# Saving data to a JSON file
with open('new_data.json', 'w') as file:
json.dump(data, file)
Excel Files:
- Excel files are widely used for storing tabular data. The
pandas
the library provides functions to read and write Excel files using theread_excel()
andto_excel()
methods.
Here’s an example:
import pandas as pd
data = pd.read_excel('data.xlsx')
# Saving data to an Excel file
data.to_excel('new_data.xlsx', index=False)
It’s important to choose the appropriate method for loading and saving data based on the specific data format and requirements. Different libraries provide different functionalities and options for handling various data types.
Pandas, in particular, is a powerful library that offers extensive support for loading, manipulating, and saving data in different formats.
15. How do you perform data visualization in Python?
data visualization is a crucial aspect of data analysis and plays a vital role in understanding and communicating insights effectively. Python provides several libraries for data visualization, each with its own strengths and capabilities.
Here are some popular libraries used for data visualization in Python:
Matplotlib:
- Matplotlib is a widely used plotting library in Python. It provides a flexible and extensive set of tools for creating various types of plots, including line plots, scatter plots, bar plots, histograms, and more. Matplotlib provides a high degree of customization, allowing users to control almost every aspect of the plot.
Seaborn:
- Seaborn is a statistical data visualization library that builds on top of Matplotlib. It provides a higher-level interface and offers visually appealing, pre-defined themes and color palettes. Seaborn is particularly useful for creating statistical plots, such as box plots, violin plots, heatmaps, and categorical plots.
Plotly:
- Plotly is a versatile library that offers interactive and web-based visualizations. It provides a range of plots, including basic charts, 3D plots, and maps. Plotly visualizations can be embedded in web applications and Jupyter notebooks, allowing for interactive exploration and sharing of data insights.
Pandas:
- Pandas, in addition to its data manipulation capabilities, also provides basic data visualization functionality. It uses Matplotlib under the hood and allows for quick plotting of data directly from Pandas DataFrames. This integration makes it convenient for exploratory data analysis and quick visualizations.
Bokeh:
- Bokeh is another library for interactive visualizations. It focuses on creating interactive and visually appealing plots that can be displayed in web browsers. Bokeh offers various interactive tools for exploring and interacting with data, making it suitable for building interactive dashboards and applications.
When performing data visualization in Python, it’s important to select the appropriate library based on the specific requirements of the task and the desired style of visualization.
16. How do you debug Python code?
debugging is an essential skill for any programmer, as it helps identify and fix errors or issues in the code. In Python, there are several techniques and tools available to debug code effectively.
Here are some common approaches to debugging Python code:
Print Statements:
- One of the simplest and most commonly used debugging techniques is to insert print statements in the code. By strategically placing print statements at various points in the code, you can print the values of variables or specific messages to understand the flow and behavior of the program during execution. This can help identify where the issue is occurring and provide insight into the state of the program.
IDE Debugging Tools:
- Integrated Development Environments (IDEs) such as PyCharm, Visual Studio Code, and PyDev offer powerful debugging tools. These tools provide features like breakpoints, stepping through code, inspecting variables, and evaluating expressions during runtime. IDEs often have a user-friendly interface for debugging, making it easier to navigate and analyze code execution.
Logging:
- Another effective debugging technique is using logging to record important information during program execution. The
logging
module in Python allows you to define log levels, log messages, and specify where the logs should be saved (e.g., console, file). By strategically placing logging statements, you can gather relevant information about the program’s behavior and troubleshoot issues.
Tesere are some of the many Python questions that you could be asked in your interview. The best way to prepare for these questions is to practice writing Python code and familiarize yourself with the different Python libraries.
Summary
In this blog post, we explored some essential Python questions that every fresher in the field of machine learning engineering should be familiar with.
Also, Read
Happy Learning And Keep Learning…
Thank You…