Hello Learners…
Welcome to my blog…
Table Of Content:
- Introduction
- What is grammar correction?
- Grammar Correction models from HuggingFace
- Save the pre-trained model on my local system
- Summary
Introduction:
In this post, we create a simple grammar correction web app using the python streamlit library and deep learning models from huggingface transformers. this web app corrects our grammar and gives a response with the corrected grammar text.
What is Grammar Correction?
Grammar Correction corrects the grammatical errors in any sentence or paragraph.
Grammar Correction models from HuggingFace
HuggingFace is an open-source deep learning models community that provides state-of-the-art models through transformers API.
This is the URL for the Grammar Correction Models By HuggingFace:
https://huggingface.co/vennify/t5-base-grammar-correction
Save the pre-trained model on my local system
To use a pre-trained model it’s a good practice to download that model to the local system and then use it. we can use it directly without downloading it. we download it on our local system first then we use it.
Here we used this model:https://huggingface.co/vennify/t5-base-grammar-correction
To save the pre-trained model on our local system we run the code below:
To run the given code we need to install pytorch and transformers using pip so we first install both
pip install torch
pip install transformers
After Installation, We create a save_pretrained.py file and paste the below code into that file
from transformers import AutoTokenizer, AutoModelForSeq2SeqLM
tokenizer = AutoTokenizer.from_pretrained("vennify/t5-base-grammar-correction")
model = AutoModelForSeq2SeqLM.from_pretrained("vennify/t5-base-grammar-correction")
tokenizer.save_pretrained("t5-base-grammar-correction")
model.save_pretrained("t5-base-grammar-correction")
Now We run the save_pretrained.py file.
python3 save_pretrained.py

It will create a t5-base-grammar-correction directory in the current directory and save all required files into this.

Now, we create a web application interface using the streamlit library.
For this first, we have to install streamlit and happytransformer using pip:
pip install streamlit
pip install happytransformer
After installation of both the above libraries now we create a streamlit_app.py file and paste the below code into that file.
import streamlit as st
from happytransformer import HappyTextToText, TTSettings
st.title("Grammar Correction")
def main():
try:
text=st.text_input("Enter your text")
if st.button("Click Here"):
st.write("Your Text: ",text)
if text :
happy_tt = HappyTextToText("T5", "t5-base-grammar-correction")
args = TTSettings(num_beams=5, min_length=1)
result = happy_tt.generate_text("grammar: "+text, args=args)
st.write("Corrected Text: ",result.text)
else:
st.error("Please enter your text")
except Exception as e:
st.error({"errror":str(e)})
main()
Now run the streamlit_app.py file
streamlit run streamlit_app.py
You get an URL: http://localhost:8501
Open It in Browser if not opened automatically. you can see the below interface.

Now enter the text, click the button, and see the results.

Here you can see our entered text and corrected text.
Here is the second example
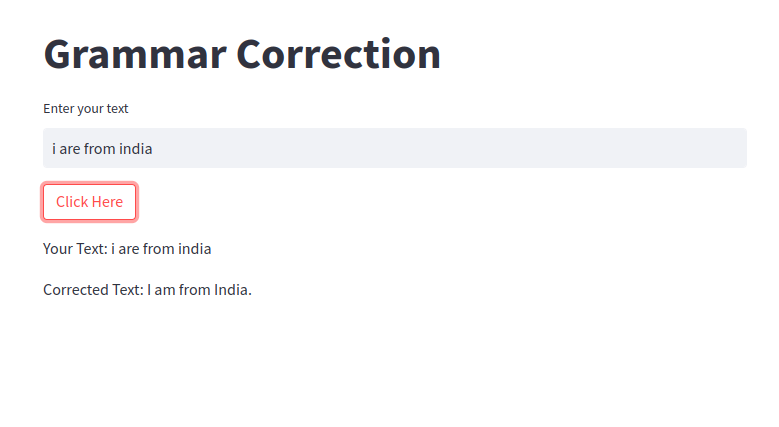
Summary
This is the basic Grammar Correction web app using Python’s streamlit library and transformers API by the huggingface community. you can use this model for your normal grammatical error correction task.it is a good way to start with NLP(Natural language Processing).
I hope you like the blog…
Happy Learning And Keep Learning…
Thank You…
1 thought on “Create Grammar Correction WebApp Using Python And HuggingFace”