Hello Learners…
Welcome To My Blog…
Table Of Contents
- Introduction
- What is an API?
- Why use an API?
- What is FastAPI?
- FastAPI Installation
- Write First Simple Python Program To Create FastAPI
- Summary
- References
Introduction
In this post, we discuss FastAPI Full Tutorial For Data Science, where we try to understand how FastAPI works and how we can implement it in our projects.
What is an API?
API stands for application program interface. which will provide an interface to work with our code or program to do some specific task.
Why use an API?
Api is a communicator between the front-end and back-end or we can say that API is helpful to use one program from another program.
API is used to provide web services or HTTP web services.
Suppose a web developer making a website and on that website, we want to integrate Machine learning but a web developer doesn’t know anything about machine learning, they should only know about API.
If they know API they can integrate machine learning without knowing how it works. they can directly use The API in the website and the website is good to go with machine learning also.
there are some important things we know before the use an API.
- What API should we use?
- How to use that API?
- What are the inputs?
- What are the outputs?
Examples we use in our daily life.
- Google text to speech
Advantages Of An API
- Increase Productivity
- Save Cost
- Enhance the customer experience
- Collects data for analysis
- Build new product capabilities
What career path can we follow after completion of the full tutorial of FastAPI?
- Web developer
- Software Developer
- Python Developer
- Java Developer
- Full stack Developer
- Front-end Developer
- Back-end Developer
- Web service provider
- Web services tool provider
- Create our own tools and sell them
API extends the capabilities of any project, making an API is part of development if you are a developer then you should know how to make an API and how to use the API.
After learning FastAPI we were able to learn a to z of a machine learning process of a project building process.
the person who has to be able to understand front and back and able to communicate with both using an API.
Even if it is MySQL, Python, Java, CSS, or whatever we use.
FastAPI Full Tutorial For Data Science
What is FastAPI?
FastAPI is a modern, fast, high-performance web application framework for building APIs in python3.6+ using Python-type hints.
Python type hints is a standard library for using type hints. we will learn more about type hints further.
FastAPI is a framework for building restful APIs. not all the HTTP APIs are restful.
There are certain architectural conditions that apply to be restful.
many big companies like Uber, Microsoft, and Netflix are building their own application with the help of FastAPI.
FastAPI Installation
First, we have to install FastAPI using pip.
pip install fastapi
we have to install uvicorn to run FastAPI Server using the pip command.
pip install "uvicorn[standard]"
Write First Simple Python Program To Create FastAPI
Create a hello_world.py file and paste the below code into this file.
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def hello():
return {"hello":"world"}
To run the hello_world.py file open the terminal and enter the command.
uvicorn hello_world:app --reload
reload used for automatic reloading our app on changing something in code.
when we run the command we get a localhost URL: http://127.0.0.1:8000
Open it in the browser and we can see the output of the FastAPI.
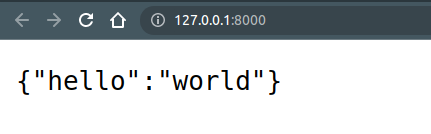
Path Operation Function
By default path is “/“. and it will call that function that is on this path.
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def hello():
return 4
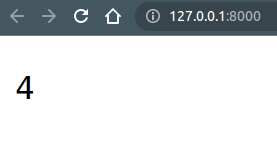
Path Parameter
Here we can pass any parameter in our path and it takes as an item and return it on the web front-end.
from fastapi import FastAPI
app =FastAPI()
@app.get("/")
def hello():
return "hello"
#Path Paremeter
@app.get("/{item}")
def show_item(item):
return {"Items":item}
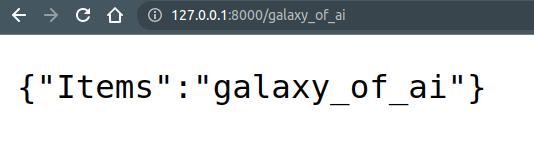
Here hello() function is the default one and show_item() is the path parameter with items.
URL : http://127.0.0.1:8000/
- output: “hello”
URL : http://127.0.0.1:8000/galaxy_of_ai
- output: {“item”:”galaxy_of_ai”}
Variable item takes an apple as a variable value. This is what the path parameter looks likes.
Python Parameter With Type Hints
What are type hints in Python?
Type hints are additional syntax in which we can provide a type of a variable in Python.
Python does not provide us to define the type of a variable. when we create a variable in Python we do not need to define its type of it. In other programming languages, we need to provide the type of a variable.
Why type hints are important?
In Python, we declare variables as below.
# Variable declaration in python
i=10
There is no problem with that, but if we define variables with their types it gives us additional support for editor and validation support.
here we define the type of our variable item as a string. anything we pass in our URL is considered a string.
from fastapi import FastAPI
app =FastAPI()
@app.get("/")
def hello():
return "hello"
@app.get("/{item}")
def show_item(item:str):
return {"Items":item}
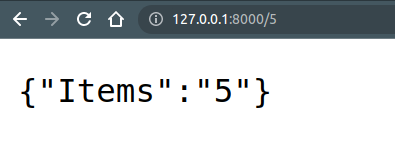
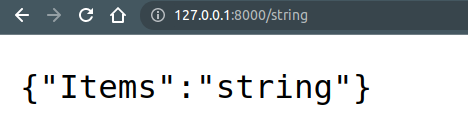
URL : http://127.0.0.1:8000/5
- Output: {“Items”: “5”}
URL : http://127.0.0.1:8000/string
- Output: {“Items”: “string”}
Another example of PythonType hints with FastAPI.
from fastapi import FastAPI
app =FastAPI()
@app.get("/")
def hello():
return "hello"
@app.get("/{item}")
def show_item(item:int):
return {"Items":item}

URL : http://127.0.0.1:8000/hello_all
- Output: {“detail”:[{“loc”:[“path”, “item”], “msg”: “value is not a valid integer”, “type”: “type_error.integer”}]}
Here we define the item type as an int but we are passing str(hello_all) in our URL that’s why we are getting an error.
Python Enumeration With FastAPI
It is not necessary to learn enumeration for FastAPI but it’s good to have knowledge of enum.
Here is the example
from enum import Enum
from fastapi import FastAPI
class Fruits(str, Enum):
orange = "orange"
apple = "apple"
grapes = "grapes"
app = FastAPI()
@app.get("/fruits/{fruit_name}")
def get_fruit(fruit_name : Fruits):
if fruit_name == fruit_name.orange:
return {"Fruit_name matched": fruit_name}
if fruit_name.value == "apple":
return {"Fruit_name matched": fruit_name}
return {"Fruit Name":fruit_name}
Query parameter
when we declare a Function Parameter that is not part of the Path parameter they are automatically interpreted as a query parameter.
Example:1
from fastapi import FastAPI
app =FastAPI()
# a and b are function parameter
def add(a,b):
return a+b
# item is a path parameter
@app.get("/{item}")
def show_item(item:int):
return {"Items":item}
# name and weight are query parameter
@app.get("/fruit")
def fruits(name:str,weight:str):
return {"fruit_name":name,"fruit_weight":weight}
Now we have to send data to the query parameter first run the above code and in the URL add the query parameter as below
URL : http://127.0.0.1:8000/fruit/?name=apple&weight=12kg
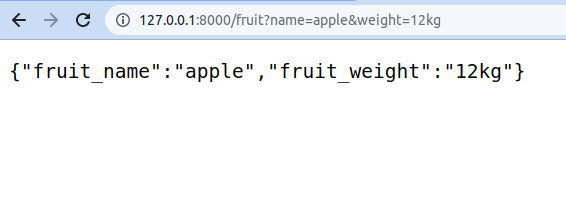
This is how we can pass query parameters if it is not path parameter.
Example:2
from fastapi import FastAPI
app =FastAPI()
@app.get("/fruit")
def fruits(count:int,weight:int):
return {"fruit_count":count,"fruit_weight":weight}
URL : http://127.0.0.1:8000/fruit/?count=56&weight=124
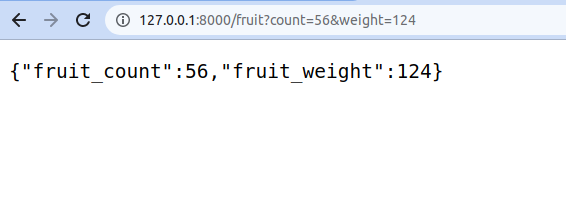
Query Parameter takes a parameter as a string but when it goes to FastAPI it converts it to data types that we are given.
Here is in the URL count and height is a string but as output, we got an integer because we define its type.
Example:3
from fastapi import FastAPI
app =FastAPI()
@app.get("/fruit")
def fruits(count:int=10,weight:int=25):
return {"fruit_count":count,"fruit_weight":weight}
URL : http://127.0.0.1:8000/fruit
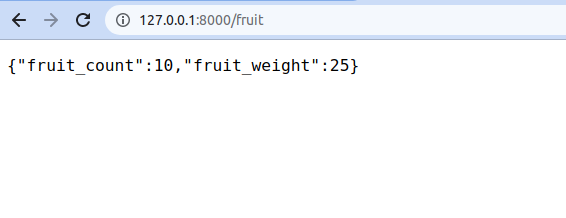
Without a query parameter, it is also working because we can define the default value of the query parameter. This is how we can set the default query parameter value.
Multiple path and query parameter
Example:1
from fastapi import FastAPI
app =FastAPI()
@app.get("/")
def hello():
return "hello"
@app.get("/fruit/{fruit_name}/count/{fruit_count}")
def fruits(fruit_name:str,fruit_count:str):
return {"fruit_name":fruit_name,"fruit_count":fruit_count}
URL : http://127.0.0.1:8000/fruit/apple/count/15
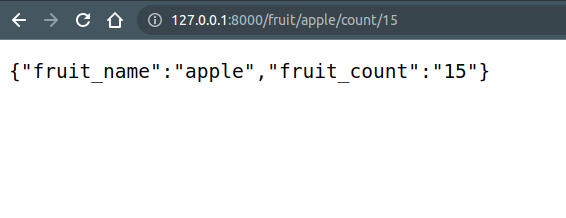
Example:2
from fastapi import FastAPI
app =FastAPI()
@app.get("/")
def hello():
return "hello"
@app.get("/fruit/{fruit_name}/count/{fruit_count}")
def fruits(total_weight:int,fruit_name:str,fruit_count:int=45):
return {"fruit_name":fruit_name,"fruit_count":fruit_count,"total_weight":total_weight}
URL : http://127.0.0.1:8000/fruit/apple/count/15?total_weight=45
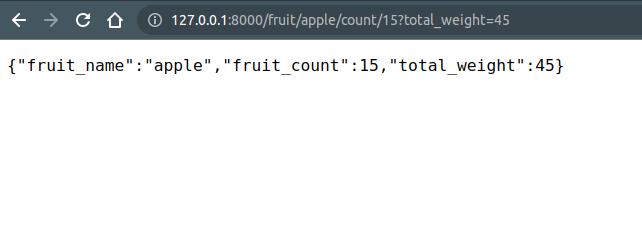
Summary
This is all about FastAPI which is required for the data scientist or machine learning engineer.
Also refer to this post,
3 thoughts on “FastAPI Full Tutorial For Data Science”