Hello Learners…!!!
Welcome to my blog.
Table Of Contents
- Introduction
- Full Stack Data Science Journey: Basic Python Programming – 42
- Summary
- Reference
Introduction:
This is the the part of Full Stack Data Science Journey Full Stack Data Science Journey: Basic Python Programming.
Full Journey: https://galaxyofai.com/full-stack-data-science-journey-introduction-1/
Full Stack Data Science Journey: Basic Python Programming – 42
Here I share some basic python programming questions that help you to improve your logic and programming skills.
Here are some questions:
- Write a Python program to print “Hello Python”
- Write a Python program to do arithmetical operations addition and division.
- Write a Python program to find the area of a triangle
- Write a Python program to swap two variables
- Write a Python program to generate a random number
Try it yourself and then compare it with our logic.
So let’s try to solve these questions, We write our logic here but every question can be solved in many ways so find your best way to solve these programming questions.
1. Write a Python program to print “Hello Python”
# 1. Write a Python program to print "Hello Python"?
print("Hello Python")

2. Write a Python program to do arithmetical operations addition and division.
# 2. Write a Python program to do arithmetical operations addition and division.?
def addition_num(a,b):
return a+b
def division_num(a,b):
return a/b
try:
a=int(input("enter first value"))
b=int(input("enter second value"))
choice=(input("enter + for addition and / for division"))
if choice=="+":
print(addition_num(a,b))
elif choice=="/":
print(division_num(a,b))
else:
print("wrong choice..")
except Exception as e:
print("Exception occured:" ,str(e))
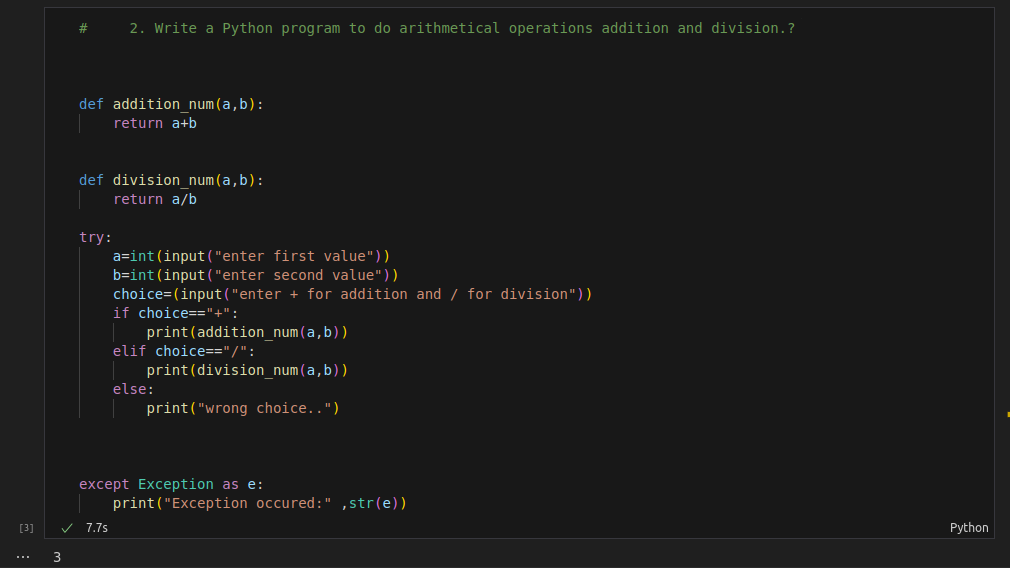
3. Write a Python program to find the area of a triangle
This Python program is designed to calculate and print the area of a triangle.
It does so by taking user input for the base and height of the triangle and then applying the formula for the area of a triangle, which is (1/2) * base * height.
# 3. Write a Python program to find the area of a triangle?
try:
base=float(input("enter value of base"))
height=float(input("enter value of height"))
triangle_area=(1/2)*base*height
print(triangle_area)
except Exception as e:
print("Exception occured..",str(e))

4. Write a Python program to swap two variables
# 4. Write a Python program to swap two variables?
try:
a=int(input("enter value of a"))
b=int(input("enter value of b"))
print("value of a is ",a)
print("value of b is ",b)
a,b=b,a
print("*******After swap***************")
print("value of a is ",a)
print("value of b is ",b)
except Exception as e:
print("Exception occured",str(e))

5. Write a Python program to generate a random number
This Python program generates and prints a random number between 0 (inclusive) and 1000 (exclusive). It uses the NumPy library to accomplish this task.
# 5. Write a Python program to generate a random number?
from numpy import random
x = random.randint(1000)
print(x)

So, when we run this program, it will produce a random integer between 0 and 999 (inclusive), and the value of that random number will be printed to the console.
Each time we run the program, we may get a different random number within this range.
Summary
we’ve explored five fundamental Python programs that serve as building blocks for your journey into the world of programming. These programs cover essential concepts and techniques that will help you develop a strong foundation in Python.
Happy Learning And Keep Learning…
Thank You…